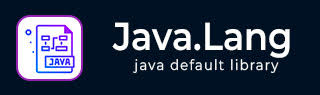
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Integer compareTo() method
Description
The Java Integer compareTo() method compares two Integer objects numerically.
Declaration
Following is the declaration for java.lang.Integer.compareTo() method
public int compareTo(Integer anotherInteger)
Parameters
anotherInteger − This is the Integer to be compared.
Return Value
This method returns the value 0 if this Integer is equal to the argument Integer, a value less than 0 if this Integer is numerically less than the argument Integer and a value greater than 0 if this Integer is numerically greater than the argument Integer.
Exception
NA
Comparing two Positive Integer Objects Example
The following example shows the usage of Integer compareTo() method to compare two integer objects. We've created two Integer variables and assigned them Integer objects created using a positive int values. Then using compareTo() method, we're comparing the integer objects.
package com.tutorialspoint; public class IntegerDemo { public static void main(String[] args) { // compares two Integer objects numerically Integer obj1 = new Integer("25"); Integer obj2 = new Integer("10"); int retval = obj1.compareTo(obj2); if(retval > 0) { System.out.println("obj1 is greater than obj2"); } else if(retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
obj1 is greater than obj2
Comparing two Negative Integer Objects Example
The following example shows the usage of Integer compareTo() method to compare two integer objects. We've created two Integer variables and assigned them Integer objects created using a negative int values. Then using compareTo() method, we're comparing the integer objects.
package com.tutorialspoint; public class IntegerDemo { public static void main(String[] args) { // compares two Integer objects numerically Integer obj1 = new Integer("-25"); Integer obj2 = new Integer("-10"); int retval = obj1.compareTo(obj2); if(retval > 0) { System.out.println("obj1 is greater than obj2"); } else if(retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
obj1 is less than obj2
Comparing two Integer Objects with Same Value Example
The following example shows the usage of Integer compareTo() method to compare two integer objects. We've created two Integer variables and assigned them Integer objects created using a same positive int values. Then using compareTo() method, we're comparing the integer objects.
package com.tutorialspoint; public class IntegerDemo { public static void main(String[] args) { // compares two Integer objects numerically Integer obj1 = new Integer("25"); Integer obj2 = new Integer("25"); int retval = obj1.compareTo(obj2); if(retval > 0) { System.out.println("obj1 is greater than obj2"); } else if(retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
Output
Let us compile and run the above program, this will produce the following result −
obj1 is equal to obj2
To Continue Learning Please Login