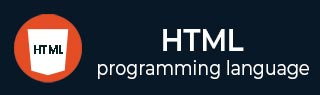
- HTML Tutorial
- HTML - Home
- HTML - Introduction
- HTML - Editors
- HTML - Basic Tags
- HTML - Elements
- HTML - Attributes
- HTML - Headings
- HTML - Paragraphs
- HTML - Fonts
- HTML - Blocks
- HTML - Style Sheet
- HTML - Formatting
- HTML - Quotations
- HTML - Comments
- HTML - Colors
- HTML - Images
- HTML - Image Map
- HTML - Iframes
- HTML - Phrase Elements
- HTML - Meta Tags
- HTML - Classes
- HTML - IDs
- HTML - Backgrounds
- HTML Tables
- HTML - Tables
- HTML - Headers & Caption
- HTML - Table Styling
- HTML - Table Colgroup
- HTML - Nested Tables
- HTML Lists
- HTML - Lists
- HTML - Unordered Lists
- HTML - Ordered Lists
- HTML - Definition Lists
- HTML Links
- HTML - Text Links
- HTML - Image Links
- HTML - Email Links
- HTML Color Names & Values
- HTML - Color Names
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML Forms
- HTML - Forms
- HTML - Form Attributes
- HTML - Form Control
- HTML - Input Attributes
- HTML Media
- HTML - Video Element
- HTML - Audio Element
- HTML - Embed Multimedia
- HTML Header
- HTML - Head Element
- HTML - Adding Favicon
- HTML - Javascript
- HTML Layouts
- HTML - Layouts
- HTML - Layout Elements
- HTML - Layout using CSS
- HTML - Responsiveness
- HTML - Symbols
- HTML - Emojis
- HTML - Style Guide
- HTML Graphics
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - Drag & Drop API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web Storage
- HTML - Server Sent Events
- HTML Miscellaneous
- HTML - MathML
- HTML - Microdata
- HTML - IndexedDB
- HTML - Web Messaging
- HTML - Web CORS
- HTML - Web RTC
- HTML Demo
- HTML - Audio Player
- HTML - Video Player
- HTML - Web slide Desk
- HTML Tools
- HTML - Velocity Draw
- HTML - QR Code
- HTML - Modernizer
- HTML - Validation
- HTML - Color Picker
- HTML References
- HTML - Cheat Sheet
- HTML - Tags Reference
- HTML - Attributes Reference
- HTML - Events Reference
- HTML - Fonts Reference
- HTML - ASCII Codes
- ASCII Table Lookup
- HTML - Color Names
- HTML - Entities
- MIME Media Types
- HTML - URL Encoding
- Language ISO Codes
- HTML - Character Encodings
- HTML - Deprecated Tags
- HTML Resources
- HTML - Quick Guide
- HTML - Useful Resources
- HTML - Color Code Builder
- HTML - Online Editor
HTML - id Attribute
HTML id attribute is used to uniquely identify an element within an HTML document, allowing for targeted styling, scripting, and linking.
This attribute is frequently used for style, managing events, and changing the structure of documents. In order to create interactive and dynamic web pages, it gives developers the ability to target particular parts and provide them specialized behavior and appearance.
Syntax
<tag id = ‘id_name’></tag>
Where id_name can be any variable name of your choice.
Applies On
Id attribute is a global attribute, which means it is supported by almost all HTML tags. However structural tags like <html>, <head> does not support id tag.
Example of HTML id Attribute
Below examples will illustrate the HTML id attribute, where and how we should use this attribute!
Use id Attribute for Text Manipulation
In this example we will create a textual content and manipulate the text through JavaScript, by selecting the element by defined id.
<!Doctype html> <html> <body> <h3>HTML id Attribute</h3> <strong id="myId">Tutorialspoint</strong> <p> Click this <button onclick="changeElement()">Button</button> to see the change </p> <script> function changeElement() { document.getElementById("myId").innerHTML = "Simply Easy Learning!"; } </script> </body> </html>
Access a div element using id Attribute
Considering the another scenario, where we are going to use the id attribute with the div element to apply styles to it using CSS.
<!DOCTYPE html> <html lang="en"> <head> <title>HTML id attribute</title> <style> /*access element using #id_name */ #demo { width: 310px; height: 120px; background-color: green; align-items: left; border-radius: 10px; } #demo p { color: white; letter-spacing: 1px; text-align: center; } </style> </head> <body> <!-- Example of HTML 'id' attribute --> <strong>HTML 'id' attribute</strong> <div id="demo"> <p> HTML id attribute is used to uniquely identify an element within an HTML document, allowing for targeted styling, scripting, and linking. </p> </div> </body> </html>
Using id as a selector to Style form
Let's look into the another example, where we are going to use the id attribute with the form element to style a login form with the help of CSS.
<!DOCTYPE html> <html lang="en"> <head> <title>HTML id attribute</title> <style> /*access element using #id_name */ #myForm { width: 300px; height: 210px; background-color: aqua; border-radius: 10px; text-align: center; } #myForm h1 { text-align: center; font-family: sans-serif; margin-top: 5px; } #myForm input { padding: 8px; margin: 5px 0px; } #myForm button { width: 105px; padding: 8px; } </style> </head> <body> <!-- Example of HTML 'id' attribute --> <p>Example of HTML 'id' attribute</p> <form id='myForm'> <h1>Login</h1> <br> Username: <input type="text"> <br> Password: <input type="password"> <br> <button>Login</button> </form> </body> </html>
Difference between id and class in HTML
In HTML, the id attribute uniquely identifies a single element on a page, making it useful for targeting with CSS and JavaScript, and it must be unique within the document. The class attribute, on the other hand, can be applied to multiple elements, allowing for the grouping of elements that share common styles or behaviors.
<!DOCTYPE html> <html lang="en"> <head> <title>Difference between id and class</title> <style> /* ID selector */ #header { background-color: blue; color: white; padding: 10px; } /* Class selector */ .button { background-color: green; color: white; padding: 5px 10px; margin: 5px; } </style> </head> <body> <!-- Unique ID for the header --> <div id="header"> This is the header </div> <!-- Shared class for buttons --> <div class="button"> Button 1 </div> <div class="button"> Button 2 </div> </body> </html>
Supported Browsers
Attribute | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
id | Yes | Yes | Yes | Yes | Yes |
To Continue Learning Please Login