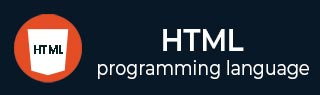
- HTML Tutorial
- HTML - Home
- HTML - Introduction
- HTML - Editors
- HTML - Basic Tags
- HTML - Elements
- HTML - Attributes
- HTML - Headings
- HTML - Paragraphs
- HTML - Fonts
- HTML - Blocks
- HTML - Style Sheet
- HTML - Formatting
- HTML - Quotations
- HTML - Comments
- HTML - Colors
- HTML - Images
- HTML - Image Map
- HTML - Iframes
- HTML - Phrase Elements
- HTML - Meta Tags
- HTML - Classes
- HTML - IDs
- HTML - Backgrounds
- HTML Tables
- HTML - Tables
- HTML - Headers & Caption
- HTML - Table Styling
- HTML - Table Colgroup
- HTML - Nested Tables
- HTML Lists
- HTML - Lists
- HTML - Unordered Lists
- HTML - Ordered Lists
- HTML - Definition Lists
- HTML Links
- HTML - Text Links
- HTML - Image Links
- HTML - Email Links
- HTML Color Names & Values
- HTML - Color Names
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML Forms
- HTML - Forms
- HTML - Form Attributes
- HTML - Form Control
- HTML - Input Attributes
- HTML Media
- HTML - Video Element
- HTML - Audio Element
- HTML - Embed Multimedia
- HTML Header
- HTML - Head Element
- HTML - Adding Favicon
- HTML - Javascript
- HTML Layouts
- HTML - Layouts
- HTML - Layout Elements
- HTML - Layout using CSS
- HTML - Responsiveness
- HTML - Symbols
- HTML - Emojis
- HTML - Style Guide
- HTML Graphics
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - Drag & Drop API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web Storage
- HTML - Server Sent Events
- HTML Miscellaneous
- HTML - MathML
- HTML - Microdata
- HTML - IndexedDB
- HTML - Web Messaging
- HTML - Web CORS
- HTML - Web RTC
- HTML Demo
- HTML - Audio Player
- HTML - Video Player
- HTML - Web slide Desk
- HTML Tools
- HTML - Velocity Draw
- HTML - QR Code
- HTML - Modernizer
- HTML - Validation
- HTML - Color Picker
- HTML References
- HTML - Cheat Sheet
- HTML - Tags Reference
- HTML - Attributes Reference
- HTML - Events Reference
- HTML - Fonts Reference
- HTML - ASCII Codes
- ASCII Table Lookup
- HTML - Color Names
- HTML - Entities
- MIME Media Types
- HTML - URL Encoding
- Language ISO Codes
- HTML - Character Encodings
- HTML - Deprecated Tags
- HTML Resources
- HTML - Quick Guide
- HTML - Useful Resources
- HTML - Color Code Builder
- HTML - Online Editor
HTML - draggable Attribute
HTML draggable attribute is global attribute that is used to specify whether an element is draggable with mouse or not.
If this attribute is not set, its value is auto, which means drag behavior is the default browser behavior. Only links and images are by default draggable. For other elements, we have to use the draggable attribute, and the draggable attribute is often used in the drag and drop operation.
Syntax
<element draggable = "true | false" >
The values can be true or false
- true: Element can be dragged.
- false: Element cannot be dragged.
Applies On
The draggable attribute can be applied to any HTML element to make it draggable. However there are some elements that typically do not support or are not practical for dragging. For example form elements like <input>, <button> , table elements like <th>, <td>, structural elements like <head>, <title> etc does not support draggable attribute.
Example of HTML draggable Attribute
Below examples will illustrate the HTML draggable attribute, where and how we should use this attribute!
Make paragraph Draggable
In the example below, we are creating a paragraph element in HTML and make it draggable.
<!DOCTYPE html> <html> <head> <style> .drag { border: 2px solid #000; border-radius: 8px; padding: 10px; background-color: #f9f9f9; color: #333; font-family: Arial, sans-serif; font-size: 16px; cursor: grab; } .drag:active { background-color: #e0e0e0; transform: scale(1.05); cursor: grabbing; } </style> </head> <body> <p class="drag" draggable="true"> This is a draggable paragraph. You can drag this to any where you wanted. </p> </body> </html>
Drag and drop a paragraph Element
Consider another example, Here we create a draggable paragraph element that can be dragged and dropped into a designated rectangular div container, using event listeners and functions to handle the drag-and-drop actions.
<!DOCTYPE html> <html> <head> <style> #div { width: 90%; height: 100px; padding: 12px; border: 2px solid gray; } .drag { border: 2px solid #000; border-radius: 8px; padding: 10px; background-color: #f9f9f9; color: #333; font-family: Arial, sans-serif; font-size: 16px; cursor: grab; } .drag:active { background-color: #e0e0e0; transform: scale(1.05); cursor: grabbing; } </style> </head> <body> <div id="div" ondrop="drop(event)" ondragover="allowDrop(event)"> </div> <br> <p id="drag" class="drag" draggable="true" ondragstart="drag(event)"> This is a draggable paragraph. Drag this element into the rectangle box. </p> <script> function allowDrop(ev) { ev.preventDefault(); } function drag(ev) { ev.dataTransfer.setData("Text", ev.target.id); } function drop(ev) { let data = ev.dataTransfer.getData("Text"); ev.target.appendChild(document.getElementById(data)); ev.preventDefault(); } </script> </body> </html>
Supported Browsers
Attribute | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
draggable | 4.0 | 9.0 | 3.5 | 6.0 | 12.0 |
To Continue Learning Please Login