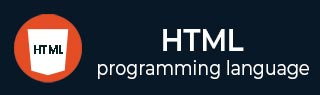
- HTML Tutorial
- HTML - Home
- HTML - Introduction
- HTML - Editors
- HTML - Basic Tags
- HTML - Elements
- HTML - Attributes
- HTML - Headings
- HTML - Paragraphs
- HTML - Fonts
- HTML - Blocks
- HTML - Style Sheet
- HTML - Formatting
- HTML - Quotations
- HTML - Comments
- HTML - Colors
- HTML - Images
- HTML - Image Map
- HTML - Iframes
- HTML - Phrase Elements
- HTML - Meta Tags
- HTML - Classes
- HTML - IDs
- HTML - Backgrounds
- HTML Tables
- HTML - Tables
- HTML - Headers & Caption
- HTML - Table Styling
- HTML - Table Colgroup
- HTML - Nested Tables
- HTML Lists
- HTML - Lists
- HTML - Unordered Lists
- HTML - Ordered Lists
- HTML - Definition Lists
- HTML Links
- HTML - Text Links
- HTML - Image Links
- HTML - Email Links
- HTML Color Names & Values
- HTML - Color Names
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML Forms
- HTML - Forms
- HTML - Form Attributes
- HTML - Form Control
- HTML - Input Attributes
- HTML Media
- HTML - Video Element
- HTML - Audio Element
- HTML - Embed Multimedia
- HTML Header
- HTML - Head Element
- HTML - Adding Favicon
- HTML - Javascript
- HTML Layouts
- HTML - Layouts
- HTML - Layout Elements
- HTML - Layout using CSS
- HTML - Responsiveness
- HTML - Symbols
- HTML - Emojis
- HTML - Style Guide
- HTML Graphics
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - Drag & Drop API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web Storage
- HTML - Server Sent Events
- HTML Miscellaneous
- HTML - MathML
- HTML - Microdata
- HTML - IndexedDB
- HTML - Web Messaging
- HTML - Web CORS
- HTML - Web RTC
- HTML Demo
- HTML - Audio Player
- HTML - Video Player
- HTML - Web slide Desk
- HTML Tools
- HTML - Velocity Draw
- HTML - QR Code
- HTML - Modernizer
- HTML - Validation
- HTML - Color Picker
- HTML References
- HTML - Cheat Sheet
- HTML - Tags Reference
- HTML - Attributes Reference
- HTML - Events Reference
- HTML - Fonts Reference
- HTML - ASCII Codes
- ASCII Table Lookup
- HTML - Color Names
- HTML - Entities
- MIME Media Types
- HTML - URL Encoding
- Language ISO Codes
- HTML - Character Encodings
- HTML - Deprecated Tags
- HTML Resources
- HTML - Quick Guide
- HTML - Useful Resources
- HTML - Color Code Builder
- HTML - Online Editor
HTML - disabled Attribute
HTML disabled is a boolean attribute that can be applied to various form elements to make them non-interactive or uneditable.
When an element is disabled, it cannot be focused, clicked, or changed by the user, and it is not included in form submission. It can be used on the following elements: <input> , <fieldset>, <button>, <textarea>, <select>, <option> and <optgroup> etc.
Syntax
<tag disabled>
Applies On
Below listed elements allow using of the HTML disabled attribute.
Element | Description |
---|---|
<input> | HTML <input> tag is used to specify the input field. |
<textarea> | HTML <textarea> tag is used to represent a multiline plain-text editing control. |
<button> | HTML <button> tag is used to embed clickable button. |
<fieldset> | HTML <fieldset> tag is used to group several controls and labels within a web form. |
<select> | HTML <select> tag is used make dropdown list to select items. |
<option> | HTML <option> tag is used name items inside dropdown list. |
<optgroup> | HTML <optgroup> is used in the select element to group together relevant option elements. |
Example of HTML disabled attribute
Bellow examples will illustrate the HTML disabled attribute, where and how we should use this attribute!
Disable input Element
In the following example, we are going to use the disabled attribute with the input field.
<!DOCTYPE html> <html lang="en"> <head> <title>HTML disabled attribute</title> </head> <body> <!--example of the 'disabled' attribute--> <p>'disabled' attribute with input element.</p> Text(disabled): <input type="text" placeholder="Disabled" disabled> <input type="text" placeholder="Not disabled"> </body> </html>
Disable button Element
Considering the another scenario, where we are going to use the disabled attribute with the button element.
<!DOCTYPE html> <html lang="en"> <head> <title>HTML disabled attribute</title> </head> <body> <!--example of the 'disabled' attribute--> <p>'disabled' attribute with button element.</p> <button disabled>Disabled</button> <button>Not Disabled</button> </body> </html>
Disable fieldset Element
Let's look at the following example, where we are going to use the disabled attribute with the fieldset element.
<!DOCTYPE html> <html lang="en"> <head> <title>HTML disabled attribute</title> </head> <body> <!--example of the 'disabled' attribute--> <p>'disabled' attribute with fieldset element.</p> <fieldset disabled> <legend>Login Form</legend> Username: <input type="text" placeholder="username"> <br> Password: <input type="password" placeholder="password"> <br> <button>Login</button> </fieldset> </body> </html>
Disable select Element
In this example will disable a whole select tag using disable attribute
<!DOCTYPE html> <html> <body> <h1>Disabled Select Element</h1> <form action="html/index.htm"> <label for="fruits">Choose a fruit:</label> <select id="fruits" name="fruits" disabled> <option value="orange">Orange</option> <option value="lemon">Lemon</option> <option value="mango">Mango</option> <option value="pineapple">Pineapple</option> </select> <br><br> <input type="submit" value="Submit"> </form> </body> </html>
Disable optgroup in Dropdown
In this example we are going to disable a group of option in select dropdown using disabled attribute
<!DOCTYPE html> <html> <body> <h1>The optgroup disabled attribute</h1> <form action="html/index.htm"> <label for="fruits">Choose a fruit:</label> <select name="fruits" id="fruits"> <optgroup label="Citrus Fruits"> <option value="orange">Orange</option> <option value="lemon">Lemon</option> </optgroup> <optgroup label="Tropical Fruits" disabled> <option value="mango">Mango</option> <option value="pineapple">Pineapple</option> </optgroup> </select> <br><br> <input type="submit" value="Submit"> </form> </body> </html>
Disable option in Dropdown
In this code we are going to use disable attribute to disable a option in dropdown list.
<!DOCTYPE html> <html> <body> <h1>Option disabled attribute</h1> <form action="html/index.htm"> <label for="fruits">Choose a car:</label> <select> <option value="volvo" disabled>Volvo</option> <option value="Maruti">Maruti</option> <option value="vw">VW</option> <option value="car98">car98</option> </select> <br><br> <input type="submit" value="Submit"> </form> </body> </html>
Disable textarea Element
In this example we disable a textarea tag using disable attribute
<!DOCTYPE html> <html> <body> <h1>The textarea disabled attribute</h1> <textarea disabled rows="7" cols="20"> At tutorialspoint you have access to multiple courses to upskill your knowledge. We offer free tutorials in all web development technologies. </textarea> </body> </html>
Script to enable a disabled Element
Following is the example, where we are going to use the disabled attribute with input tag and enable it on a button click using javascript.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Enable Text Input</title> <script> function enableInput() { document.getElementById("textInput").disabled = false; } </script> </head> <body> <h3>Click the button to enable text input</h3> <label for="textInput">Enter Text:</label> <input type="text" id="textInput" disabled> <button onclick="enableInput()">Enable Input</button> </body> </html>
Supported Browsers
Attribute | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
disabled | Yes | Yes | Yes | Yes | Yes |
To Continue Learning Please Login