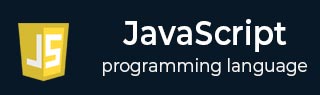
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Array toSpliced() Method
In JavaScript, the Array.toSpliced() method is used to modify an array by removing or replacing existing elements and/or adding new elements. This method is similar to the JavaScript Array.splice() method.
The toSpliced() method modifies multiple array elements: it removes the given number of elements from the array, starting at a given index, and then inserts the given elements at the same index. This method does not modify/overwrite the original array; instead, it returns a new array.
Syntax
Following is the syntax of JavaScript Array.toSpliced() method −
toSpliced(start, deleteCount, item1, item2, ..., itemN)
Parameters
This method takes the following parameters −
- start − The index at which to start changing the array.
- deleteCount (optional) − The number of elements to remove. If omitted or 0, no elements are removed.
- item1, item2, ...,itemN (optional) − The elements to add to the array at the start index.
Return value
This method returns a new array which contains the modified elements.
Examples
Example 1
In the following example, we are using the JavaScript Array toSpliced() method to remove all the array elements, starting from the index postion 2.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2); document.write(result); </script> </body> </html>
After executing the program, all the elements from index position 2 are removed.
Output
apple,banana
Example 2
If the 'deleteCount' parameter of the toSpliced() method is provided as 0, no element will be removed from the animals array.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 0); document.write(result); </script> </body> </html>
Output
As we can see the output, no element has been removed from the array.
apple,banana,cherry,dates
Example 3
The below program removes 0 (zero) elements before index position 2, and inserts the new element "Pineapple".
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 0, "Pineapple"); document.write(result); </script> </body> </html>
Output
As we can see the output, the element “Pineapple” is inserted at index postion 2 without removing any exising element.
apple,banana,Pineapple,cherry,dates
Example 4
The following program removes 0 (zero) elements before index position 2, and inserts two new elements i.e. “Pineapple” and “Grapes”.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 0, "Pineapple", "Grapes"); document.write(result); </script> </body> </html>
The elements “Pineapple” and “Grapes” are inserted at index postion 2 without removing any exising element.
Output
apple,banana,Pineapple,Grapes,cherry,dates
Example 5
In the following example, we are removing 2 elements, starting from the index position 2.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 2); document.write(result); </script> </body> </html>
After executing the program, it removed “cherry” and “dates” from the array.
Output
apple,banana
Example 6
Here, we are removing 1 element at index position 2, and inserting a new element “Grapes”.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 1, "Grapes"); document.write(result); </script> </body> </html>
After executing the program, the element “cherry” will be removed and “Grapes” will be inserted in that index.
Output
apple,banana,Grapes,dates
Example 7
In this example, we are removing 1 element at index position 2, and inserting two new elements “Grapes” and “Pineapple”.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 1, "Grapes", "Pineapple"); document.write(result); </script> </body> </html>
After executing the program, the element “cherry” will be removed and the elements “Grapes” and “Pineapple” will be inserted.
Output
apple,banana,Grapes,Pineapple,dates
Example 8
Here, we are removing 1 element from index -2 (counts from end of the array).
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(-2, 1); document.write(result); </script> </body> </html>
After executing the program, the element “cherry” will be removed.
Output
apple,banana,dates
Example 9
Here, we are removing 1 element from index -2 (counts from end of the array), and inserting two new elements “Pineapple” and “Grapes”.
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(-2, 1, "Pineapple", "Grapes"); document.write(result); </script> </body> </html>
After executing the program, the element “cherry” will be removed and “Pineapple” and “Grapes” will be removed.
Output
apple,banana,Pineapple,Grapes,dates
To Continue Learning Please Login