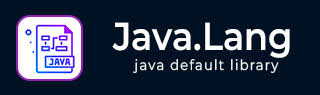
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Compiler Class
Introduction
The Java Compiler class is provided to support Java-to-native-code compilers and related services. By design, it serves as a placeholder for a JIT compiler implementation.
Note − This API is deprecated since Java 9 and is not available Java 21 onwards.
Class Declaration
Following is the declaration for java.lang.Compiler class −
public final class Compiler extends Object
Class methods
Sr.No. | Method & Description |
---|---|
1 | static Object command(Object any)
This method examines the argument type and its fields and perform some documented operation. |
2 | static boolean compileClass(Class<?> clazz)
This method compiles the specified class. |
3 | static void disable()
This method causes the Compiler to cease operation. |
4 | static boolean compileClasses(String string)
This method compiles all classes whose name matches the specified string.. |
5 | static void enable()
This method cause the Compiler to resume operation. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
Enabling a Compiler Example
The following example shows the usage of java.lang.Compiler.enable() method. In this program, we've enabled the compiler using enable() method. Then prepare a compile command using command() method. Then we've retrieved hashcode of a new Integer object created and printed the same.
package com.tutorialspoint; public class CompilerDemo { public static void main(String[] args) { // checking if compiler is enabled or not Compiler.enable(); System.out.println("Compiler Enabled..."); Compiler.command("{java.lang.Integer.*}(compile)"); Integer i = new Integer("50"); // returns a hash code value int retval = i.hashCode(); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Compiler Enabled... Value = 50
To Continue Learning Please Login