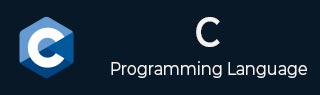
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
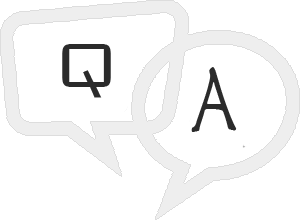
Q 1 - What is the output of the below code snippet?
#include<stdio.h> main() { int a = 5, b = 3, c = 4; printf("a = %d, b = %d\n", a, b, c); }
Answer : A
Explanation
a=5,b=3 , as there are only two format specifiers for printing.
Q 2 - What will be printed for the below statement?
#include<stdio.h> main() { printf("%d",strcmp("strcmp()","strcmp()")); }
Answer : A
Explanation
0, strcmp return 0 if both the strings are equal
Q 3 - Identify the invalid constant used in fseek() function as ‘whence’ reference.
Answer : C
Explanation
SEEK_BEG, all the rest are valid constants defined in ‘stdio.h’
Q 4 - Choose the invalid predefined macro as per ANSI C.
Answer : D
Explanation
There is no macro define with the name __C++__, but __cplusplus is defined by ANSI)
Q 5 - What is the output of the following program?
#include<stdio.h> int main(); void main() { printf("Okay"); }
Answer : D
Explanation
It’s compile error as the declaration of main() mismatches with the definition.
Q 6 - In normalized form, if the binary equivalent of 5.375 is “0100 0000 1010 1100 0000 0000 0000 0000” then what will be the output of the program in Intel core machine?
#include<stdio.h> #include<math.h> int main () { float a = 5.375; char *p; int i; p = (char*)&a; for(i=0; i <= 3; i++) printf("%02x\n", (unsigned char)p[i]); return 0; }
Answer : B
Explanation
Depends upon the machine whether its big endian or small endian machine. Byte by byte is fetched from right end.
#include<stdio.h> #include<math.h> int main () { float a = 5.375; char *p; int i; p = (char*)&a; for(i=0; i <= 3; i++) printf("%02x\n", (unsigned char)p[i]); return 0; }
Q 7 - The correct order of mathematical operators in mathematics and computer programming,
A - Addition, Subtraction, Multiplication, Division
B - Division, Multiplication, Addition, Subtraction
Answer : B
Explanation
It is BODMAS.
Q 8 - Which of the following variable cannot be used by switch-case statement?
Answer : C
Explanation
Switch Case only accepts integer values for case label, float values can’t be accepted in case of Switch Case.
#include<stdio.h> int main () { i = 1.8 switch ( i ) { case 1.6: printf ("Case 1.6"); break; case 1.7: printf ("Case 1.7"); break; case 1.8: printf ("Case 1.8"); break; default : printf ("Default Case "); } }
Q 9 - Choose the correct order from given below options for the calling function of the code “a = f1(23, 14) * f2(12/4) + f3();”?
Answer : D
Explanation
Evaluation order is implementation dependent.
Q 10 - According to ANSI specification, how to declare main () function with command-line arguments?
A - int main(int argc, char *argv[])
B - int char main(int argc, *argv)
Answer : A
Explanation
Some time, it becomes necessary to deliver command line values to the C programming to execute the particular code when the code of the program is controlled from outside. Those command line values are called command line arguments. The command line arguments are handled by the main() function.
Declaration of main () with command-line argument is,
int main(int argc, char *argv[])
Where, argc refers to the number of arguments passed, and argv[] is a pointer array which points to each argument passed to the program.
To Continue Learning Please Login