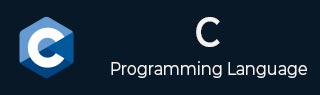
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Pointers and Arrays in C
In C programming, the concepts of arrays and pointers have a very important role. There is also a close association between the two. In this chapter, we will explain in detail the relationship between arrays and pointers in C programming.
Arrays in C
An array in C is a homogenous collection of elements of a single data type stored in a continuous block of memory. The size of an array is an integer inside square brackets, put in front of the name of the array.
Declaring an Array
To declare an array, the following syntax is used −
data_type arr_name[size];
Each element in the array is identified by a unique incrementing index, starting from "0". An array can be declared and initialized in different ways.
You can declare an array and then initialize it later in the code, as and when required. For example −
int arr[5]; ... ... a[0] = 1; a[1] = 2; ... ...
You can also declare and initialize an array at the same time. The values to be stored are put as a comma separated list inside curly brackets.
int a[5] = {1, 2, 3, 4, 5};
Note: When an array is initialized at the time of declaration, mentioning its size is optional, as the compiler automatically computes the size. Hence, the following statement is also valid −
int a[] = {1, 2, 3, 4, 5};
Example: Lower Bound and Upper Bound of an Array
All the elements in an array have a positional index, starting from "0". The lower bound of the array is always "0", whereas the upper bound is "size − 1". We can use this property to traverse, assign, or read inputs into the array subscripts with a loop.
Take a look at this following example −
#include <stdio.h> int main(){ int a[5], i; for(i = 0; i <= 4; i++){ scanf("%d", &a[i]); } for(i = 0; i <= 4; i++){ printf("a[%d] = %d\n",i, a[i]); } return 0; }
Output
Run the code and check its output −
a[0] = 712952649 a[1] = 32765 a[2] = 100 a[3] = 0 a[4] = 4096
Pointers in C
A pointer is a variable that stores the address of another variable. In C, the symbol (&) is used as the address-of operator. The value returned by this operator is assigned to a pointer.
To declare a variable as a pointer, you need to put an asterisk (*) before the name. Also, the type of pointer variable must be the same as the type of the variable whose address it stores.
In this code snippet, "b" is an integer pointer that stores the address of an integer variable "a" −
int a = 5; int *b = &a;
In case of an array, you can assign the address of its 0th element to the pointer.
int arr[] = {1, 2, 3, 4, 5}; int *b = &arr[0];
In C, the name of the array itself resolves to the address of its 0th element. It means, in the above case, we can use "arr" as equivalent to "&[0]":
int *b = arr;
Example: Increment Operator with Pointer
Unlike a normal numeric variable (where the increment operator "++" increments its value by 1), the increment operator used with a pointer increases its value by the sizeof its data type.
Hence, an int pointer, when incremented, increases by 4.
#include <stdio.h> int main(){ int a = 5; int *b = &a; printf("Address of a: %d\n", b); b++; printf("After increment, Address of a: %d\n", b); return 0; }
Output
Run the code and check its output −
Address of a: 6422036 After increment, Address of a: 6422040
The Dereference Operator in C
In C, the "*" symbol is used as the dereference operator. It returns the value stored at the address to which the pointer points.
Hence, the following statement returns "5", which is the value stored in the variable "a", the variable that "b" points to.
int a = 5; int *b = &a; printf("value of a: %d\n", *b);
Note: In case of a char pointer, it will increment by 1; in case of a double pointer, it will increment by 8; and in case of a struct type, it increments by the sizeof value of that struct type.
Example: Traversing an Array Using a Pointer
We can use this property of the pointer to traverse the array element with the help of a pointer.
#include <stdio.h> int main(){ int arr[5] = {1, 2, 3, 4, 5}; int *b = arr; printf("Address of a[0]: %d value at a[0] : %d\n",b, *b); b++; printf("Address of a[1]: %d value at a[1] : %d\n", b, *b); b++; printf("Address of a[2]: %d value at a[2] : %d\n", b, *b); b++; printf("Address of a[3]: %d value at a[3] : %d\n", b, *b); b++; printf("Address of a[4]: %d value at a[4] : %d\n", b, *b); return 0; }
Output
Run the code and check its output −
address of a[0]: 6422016 value at a[0] : 1 address of a[1]: 6422020 value at a[1] : 2 address of a[2]: 6422024 value at a[2] : 3 address of a[3]: 6422028 value at a[3] : 4 address of a[4]: 6422032 value at a[4] : 5
Points to Note
It may be noted that −
- "&arr[0]" is equivalent to "b" and "arr[0]" to "*b".
- Similarly, "&arr[1]" is equivalent to "b + 1" and "arr[1]" is equivalent to "*(b + 1)".
- Also, "&arr[2]" is equivalent to "b + 2" and "arr[2]" is equivalent to "*(b+2)".
- In general, "&arr[i]" is equivalent to "b + I" and "arr[i]" is equivalent to "*(b+i)".
Example: Traversing an Array using the Dereference Operator
We can use this property and use a loop to traverse the array with the dereference operator.
#include <stdio.h> int main(){ int arr[5] = {1, 2, 3, 4, 5}; int *b = arr; int i; for(i = 0; i <= 4; i++){ printf("a[%d] = %d\n",i, *(b+i)); } return 0; }
Output
Run the code and check its output −
a[0] = 1 a[1] = 2 a[2] = 3 a[3] = 4 a[4] = 5
You can also increment the pointer in every iteration and obtain the same result −
for(i = 0; i <= 4; i++){ printf("a[%d] = %d\n", i, *b); b++; }
The concept of arrays and pointers in C has a close relationship. You can use pointers to enhance the efficiency of a program, as pointers deal directly with the memory addresses. Pointers can also be used to handle multi−dimensional arrays.
To Continue Learning Please Login